Debugging Tips and Strategies
Debugging is the process of finding and fixing problems, or bugs, in your game. In the 1940’s one of the first programmers, a woman named Admiral Grace Hopper, was working on the Mark II computer at Harvard. She tracked down an error in her program by locating a moth that had become caught in the computer’s wiring. This led to the term “debugging” to mean the removal of bugs that cause errors in your program.
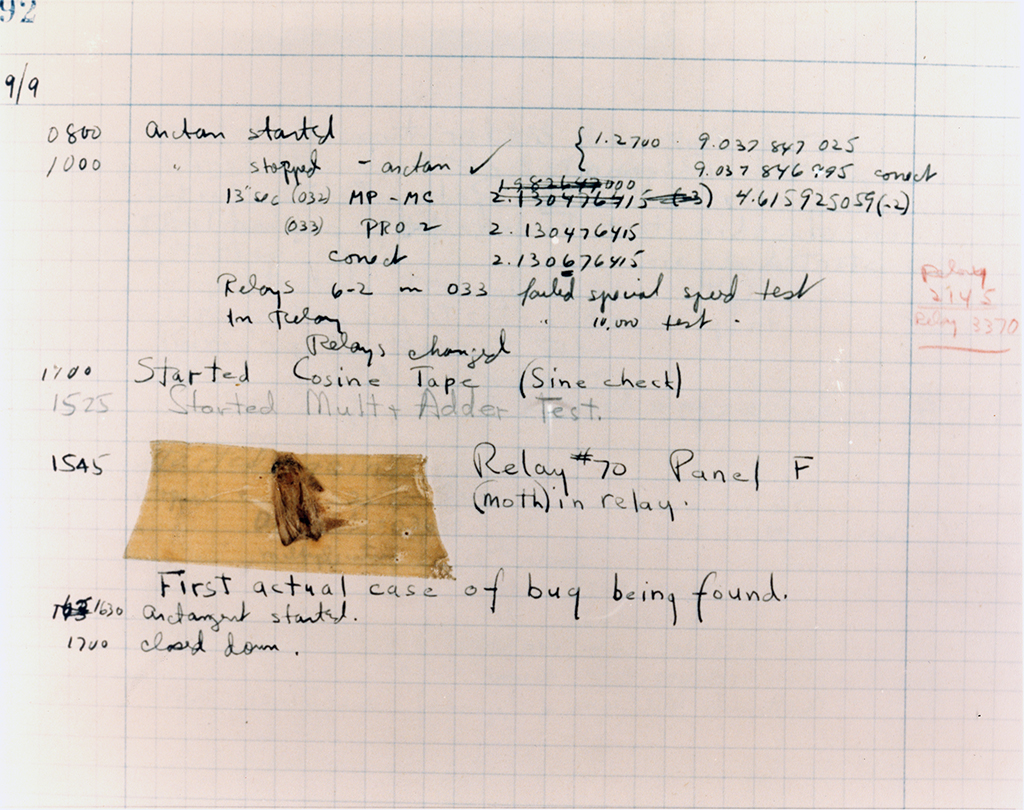
Today, you are unlikely to find actual moths in your program, but bugs are a common part of game development. In his interview, James Marr talks about the challenges of debugging and strategies to fix bugs in their games. Here are some top tips to help you when you are trying to debug your game.
Clear Your Mind
Struggling to find a solution to a bug can be frustrating. It always helps to step away for a few minutes and go clear your mind. Your brain will keep processing the problem while you do other things and when you return to your computer, the solution often presents itself. Game studios almost always have spaces for developers to play video games, read, and relax for this purpose. Try going for a walk or a bike ride and then revisit your bug.
Rubber Ducking
Sometimes talking through your problem is the best way to solve it. Rubber ducking is the process of describing out loud what you are trying to do and the problem you are encoutering to a physical rubber duck sitting on your desk. Many developers keep a duck next to their computer for this purpose, but you can just as easily borrow a friend, family member, or teacher to listen to you talk through your problem.
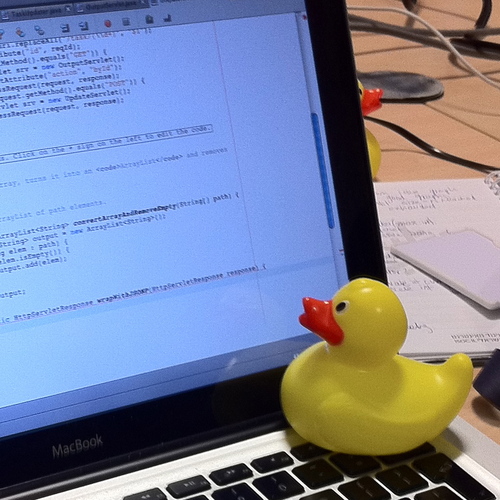
Print Debugging
When debugging, you are trying to find the exact moment where things stop working as expected. Programmers often insert commands to print messages indicating that a program is executing at a specific location, or to print out the value of a variable so you can read an confirm it contains what you expect. For example you might do something like this using the console.log()
function in Javascript:
var score = 500;
function addPoints(points)
{
console.log("score before: " + score);
score = score - points;
console.log("score after: " + score);
}
addPoints(100);
And this would then print out:
score before: 500
score after: 400
Wait a minute, the player’s score went down? You know the code that changes the score lives between the message score before: 500
and score after: 400
you can focus your attention on that code.
And sure enough, there’s a bug! The function is subtracting the points instead of adding them.
Methodically Remove Functionality
For particularly challenging bugs, learning how to methodically remove parts of your program is a very effective technique. Remove one part at a time and re-run your game to see if the bug still exists. Keep repeating this process until the bug is no longer found. The last part you removed is the likely cause of your bug. Now you can examine that code for errors and slowly re-add your removed code to confirm the bug is actually fixed.
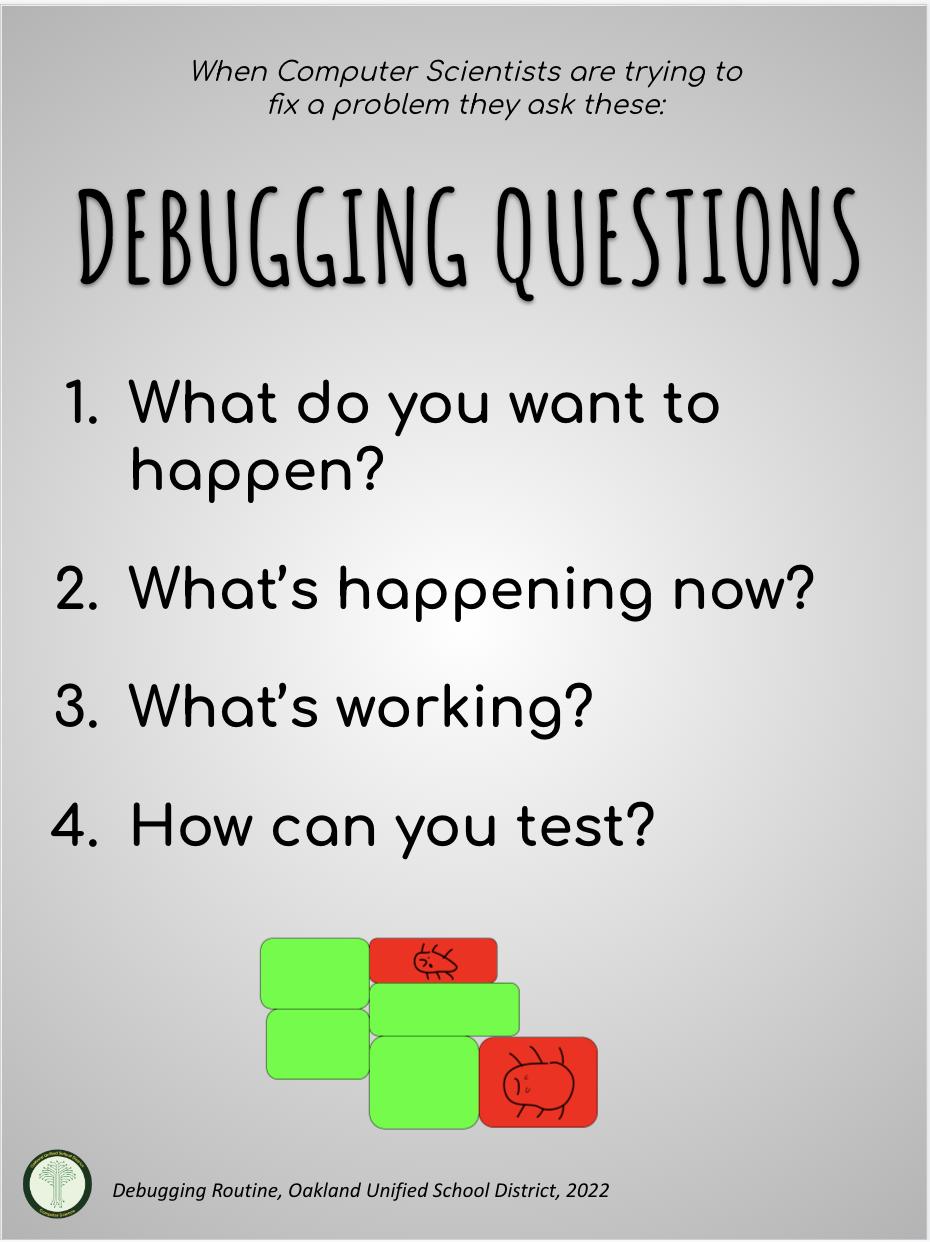